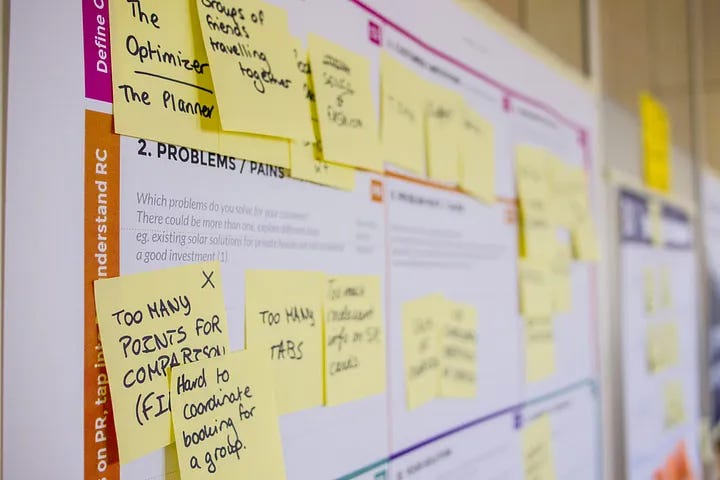
I couldn’t be more grateful when I began using a new framework like Vue.js and realized they have validation solutions. Vuelidate helps you check form input. No more reinventing the wheel.
Vuelidate
Vuelidate is a simple validation for Vue.js. It allows you to decouple it from the template. Vuelidate is dependency-free and uses a minimalistic design.
Install
Let’s get started by setting up a basic Vue.js project. Enter the following on your command terminal. Note you may need to run this with sudo.
vue create vuelidate-demo
Select the default option to get things started.
And keep rolling from there.
Finishing up you should see.
Then check your install
If that looks good you can proceed. Type the following into your terminal command.
npm install @vuelidate/core @vuelidate/validators
Then you should see this.
Now you are ready to do some coding. So let’s start with some basics.
Basics
Let’s create a simple form that uses Vuelidate.
<template>
Name:
<label>
<input v-model="name" @blur="v$.name.$touch">
<div v-if="v$.name.$error">Name field has an error.</div>
</label>
</template>
<script>
import { useVuelidate } from '@vuelidate/core'
import { required } from '@vuelidate/validators'
export default {
setup () {
return {
v$: useVuelidate()
}
},
data () {
return {
name: ''
}
},
validations () {
return {
name: { required }
}
}
}
</script>
Now when you open your browser you will see this. Prepare not to be amazed!
We are using the blur event to call the touch method. This sets the $dirty state that Vuelidate monitors. This example also uses the v-model declaration that ties to the $model object. This is used to validate the object. Check out the code here.
Built-in Validators
There are numerous built-in validators. Use the following to include them.
import { required, maxLength } from '@vuelidate/validators'
Required is a common one to use. Here is a code example.
export default {
validations () {
return {
name: { required }
}
}
}
This will check for empty arrays or strings of whitespace.
Maxlength checks for, you guessed it, the max length!
export default {
validations () {
return {
name: {
maxLength: maxLength(this.foo),
maxLengthRef: maxLength(someRef),
maxLengthValue: maxLength(10),
}
}
}
}
It works on strings, objects, or arrays. There is a lot more to choose from. Also, you can write your custom validator if these don’t solve your challenge.
Vuelidate is quite handy if you live in the Vue.js world. Validations can be quick and easy. With a little trial and error, you should find your way around in no time.