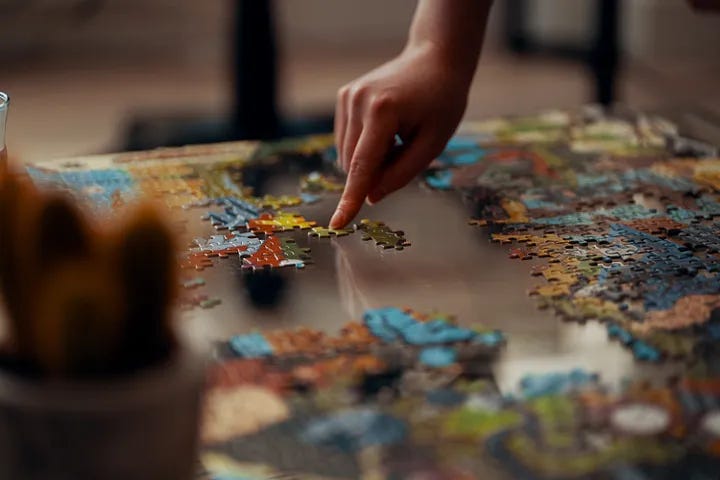
Puzzles take patience. I have tried to put them together before. I cleared the table and put them out. Then it sits barely begun. After walking by a few times I realize I should just put it away.
Crafting an application takes many pieces. We need to update some text after input. Or pass data or properties between components in our code. Today we will review some fragments in the Vue.js puzzle to help you do that.
Watchers
In life, we need to react to our surroundings. Software needs to do this too. The watch in Vue.js can perform this. In this example when a button is clicked we update information.
<script setup>
import { ref, watch } from 'vue'
const minionId = ref(1)
const minionData = ref(null)
async function fetchMinion() {
minionData.value = null
const res = await fetch(
`https://jsonplaceholder.typicode.com/todos/${minionId.value}`
)
minionData.value = await res.json()
}
fetchMinion()
watch(minionId, fetchMinion)
</script>
<template>
<p>"Don't worry, I will catch you."-Gru</p>
<p>Minion id: {{ minionId }}</p>
<button @click="minionId++" :disabled="!minionData">More Minons</button>
<p v-if="!minionData">Loading...</p>
<pre v-else>{{ minionData }}</pre>
</template>
Here is the code running in the browser. As we click the button the watcher code runs.
Components
As an experienced, some might say old!, Java developer components remind me of objects. In Java, we might import an object, in Vue.js we import a component.
<script setup>
import Sub from './Sub.vue'
</script>
<template>
<Sub />
</template>
This is the Main.vue that imports or calls the sub-component.
<template>
<h2>A Sub Component!</h2>
</template>
This is the Sub.vue component. Note the syntax to call it in the Main.vue. These two are in the same folder. You may need to alter that depending on where you place the components.
That is what you should see if you have everything working.
Props
When you have two components you may need to pass information. This can be done using props.
<script setup>
import { ref } from 'vue'
import ChildComp from './BabyBear.vue'
const greeting = ref('Mama Bear Loves you!')
</script>
<template>
<ChildComp :msg="greeting" />
</template>
Here is our MamaBear.vue which is the parent component. It is passing the greeting.
<script setup>
const props = defineProps({
msg: String
})
</script>
<template>
<h2>{{ msg || 'Where are my props?' }}</h2>
</template>
BabyBear.vue receives the props message. Note we have a default message if the props aren’t passed here. Also, the defineProps() is a compile-time macro that is automatically imported for you.
Your mileage may vary but your screenshot should match this one. Or at least be in the ballpark if you don’t check the names and spelling of the props.
We have exposed you to some Vue.js puzzle pieces. I have shared some simple examples. Now you can take them and manipulate them. Use this as a jumping-in point.