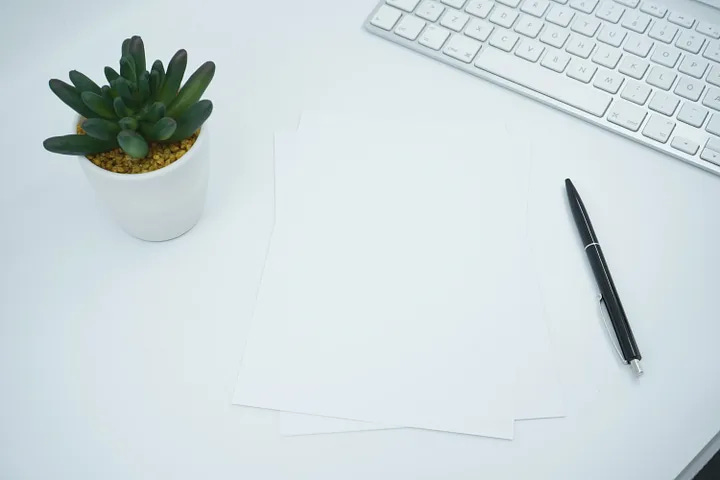
One of my first jobs working on the web was to take a paper form and create a webpage. It was quite a cumbersome process. The web technology wasn’t as robust as it is now.
We are going to review some Vue.js tools that make forms easy. For instance, the two-way bindings are a wonderful tool to use. It’s light years ahead of my early work in my career.
Form Bindings
Let’s play around with two-way bindings. Check out this simple example using a v-model component.
If we test it out you should see the text displayed on the screen as you type it.
If you don’t see it check your code. I fat-fingered the name in the v-model at first. Mistakes happen but they are part of the learning!
Conditional Rendering
Developing code requires flexibility. We need to be able to execute code for one condition or the other. The if-then syntax is ubiquitous in programming. Vue.js has it too!
When dealing with fickle values we can use the v-if and v-else. Then your code can react to new input like in the example here.
When things go through the v-if you see this.
When things go through the v-else you see this.
You will undoubtedly get to use these if you do some Vue.js programming.
List Rendering
Everyone has a list. Things to do, things to say. Here is how we render a list in Vue.js using the v-for syntax. As you can see it is great for looping over an array of objects.
When you run this example you see this below.
Each of these simple exercises helps you understand how to become productive in Vue.js. Once you see how the basics work you can move quickly to more advanced topics.
Computed Property
To react to user input we can leverage computed properties. We could filter our task list to remove completed tasks. That would look something like this.
This builds on our previous work with the List Rendering. Computed properties will come in handy for your coding in Vue.js. I have used many of them in my short time programming front-end code.
Here is the first screenshot of this example, where all values are showing:
Here is one where you hide the completed tasks.
There are numerous variations on this theme with computed properties. Depending on what your users ask for it can come in handy.
Overall, we have many tools to choose from when building applications with Vue.js. Of course, we just scratched the surface with these four items. As a coder, you will want to play around with these and use them too.