Find the best Answer to your questions with GraphQL
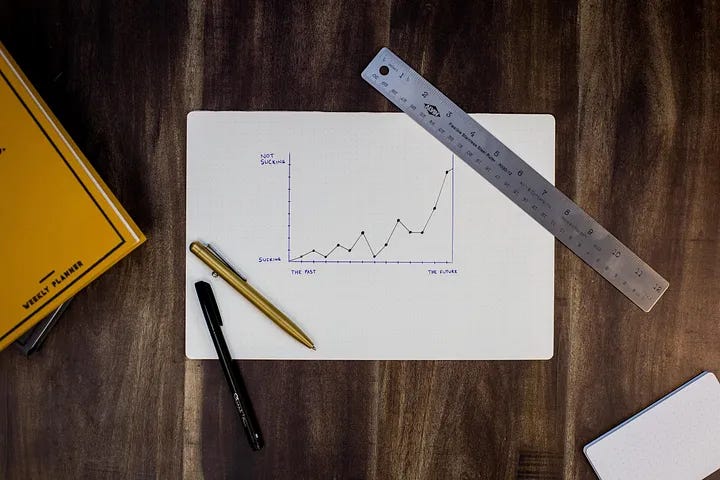
As the son of a teacher, I enjoy learning. When asked if I wanted to work on some front-end development, I said, “Yes, please.” My current work is on a Vue.js application.
It also uses GraphQL. That is a new one for me. My first thought was of SQL. I have used that for years. Structured Query Language or SQL is used for querying or finding data in a relational database.
What is GraphQL?
The word graph threw me off at first. According to their website. “GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data.” So it’s more of a tool for APIs than graphs. Perhaps APIQL wouldn’t roll off the tongue.
They say it is as easy as these three steps.
Describe your data
Ask for what you want
Get predictable results
It seems easy enough. Well, let’s see if it is that easy.
A quick note, our application is in JavaScript and uses an Apollo server. So I won’t review the many other languages and servers.
Selling points
Okay, they claim it’s easy to use. One of the other selling points is to get multiple resources in a single request. For example, you may need to get data from three systems. With GraphQL you can combine them.
What if you need to add a field? No need to version things. GraphQL can handle the changes and keep existing queries working. A big nod to backward compatibility.
When to use it
In this article, they outline when to use it. “The best scenario to use GraphQL is when you want to provide a better experience to your developers.” Hey, who doesn’t like their job to be easier?
When to not
They also point out that GraphQL has a steep learning curve. So it may take time to become proficient. Where REST is easier to learn and has a larger community support.
Data Example
Here is an example of a schema for a Patient Request and response. These are quite simple examples to share the basics you might need.
const PatientRequest = `
input PatientRequest {
patientID: String
patientName: String
insuranceCompany: String
}`;
const PatientResponse = `
input PatientResponse {
valid: Boolean
errorMsg: String
}`;
JavaScript
Using GraphQL with JavaScript is possible with GraphQL.js. As Khalil Stemmler outlines here. Here is a short example to see the basics.
const { graphql, buildSchema } = require('graphql');
// Builds a schema using the GraphQL schema language
const schema = buildSchema(`
type Query {
name: String
}
`);
// The root of our graph gives us access to resolvers for each type and field
const resolversRoot = {
hello: () => {
return 'Michael Scott!';
},
};
// Run a simple graphql query '{ hello }' and then print the response
graphql(schema, '{ hello }', resolversRoot).then((response) => {
console.log(JSON.stringify(response.data));
});
Learning path
The team at GraphQL has an extensive learning path to get you started. This is a great resource for novices like myself. You can also drill into topics that come up.
Apollo Server
There are different options you can use. Our team is using Apollo Server. You can get started here. From there you set up your project, install dependencies, and then set up your schema.
Overall, we have introduced you to some major parts of working with GraphQL. As you can see there are a few ancillary technologies. My exposure is through JavaScript but you may use other languages. Give it a try and see what you learn.