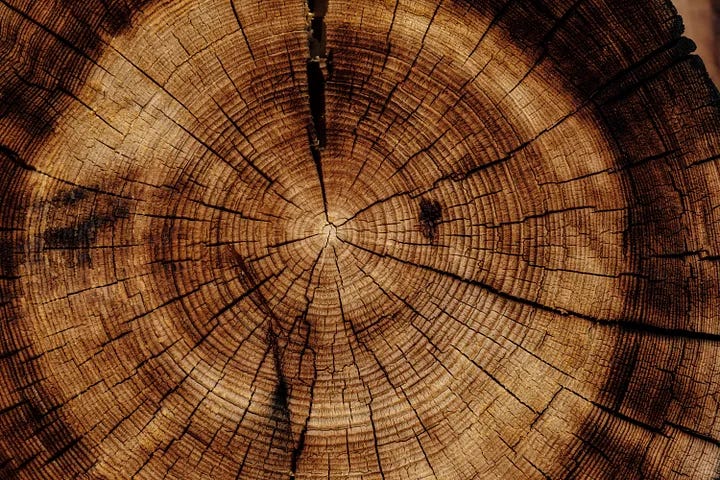
Nothing lives forever. Perhaps you remember losing your first pet or a loved one. We all have a lifecycle. Vue.js also has it too.
Lifecycle
As we learn more we need to look under the hood. To do more internal changes on a page we must manipulate the DOM. That is where the Vue.js Lifecycle comes in.
Template Ref
First off, we need to request a reference to the template. To do that we use the following example.
<input ref="input">
To learn more about them and other types check out the documentation here.
Lifecycle Hooks
Vue.js exposes many lifecycle hooks to register callbacks. The one we will use today is onMounted. To see them all look at this diagram. As a newbie, this may be a bit overwhelming. Just know it's there.
<script setup>
import { ref, onMounted } from 'vue'
const pElementRef = ref(null)
onMounted(() => {
pElementRef.value.textContent = 'Welcome to the Vue.js Lifecycle: onMounted has run'
})
</script>
<template>
<p ref="pElementRef">text</p>
</template>
In this example, we use the onMounted hook. It is used to update the message.
This gives you an idea of how to start using the Lifecycle hook with the template ref.
Comes Alive
I reached out to a mentor about the Vue.js Lifecycle. This is what he had to say:
The vue lifecycle is the place to start! It's the way to understand how a vue component comes alive (and ultimately dies). While there’s certainly a lot that happens between the arrows in the diagram, the hooks/methods available to us ( they are the outlined red boxes as created, updated, destroyed, etc) are a useful guide to understanding when things happen, and what’s available to the component at any given time.
So we need to get a firm grasp on this. That will help you see what is happening inside your application.
Composition of Components
He also mentioned we need to see how this relates to the components we use.
Understanding the basic composition of components and their use of data and events is probably a good way to see the lifecycle in action. The example code in that link shows “vanilla” vue, so while it looks different than what you’ll find in the app, the app just has some syntactic sugar available. e.g. the prop binding like v-bind:title=”post.title” in the blog example is nothing more than :title=”title” in our app, or v-on:click=”$emit(‘enlarge-text’)” becomes @click=”$emit(‘enlarge-text’)”
Essentially, if we see the big picture and the role each plays we can develop better solutions.
Don’t hurry past the Vue.js Lifecycle. This is the plumbing we need to grasp. As you develop solutions you will have to dip into this. These hooks and references give you the power you need.