Can an old Java programmer learn Javascript? Perhaps we can
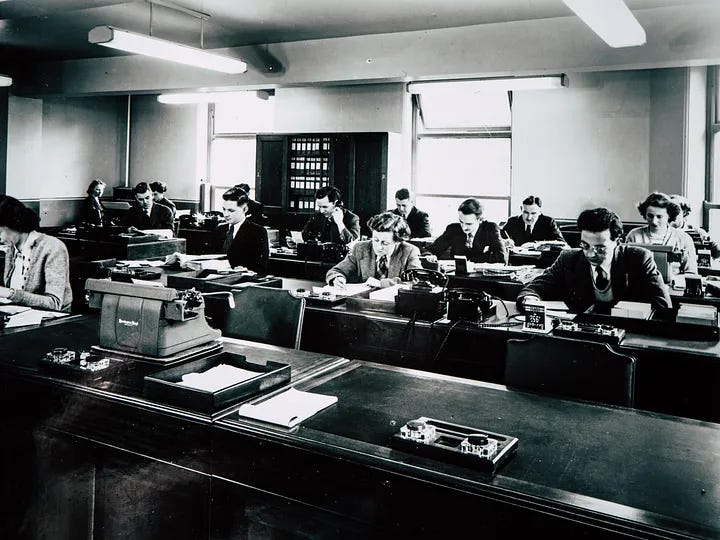
It’s no lie that Java and Javascript are often confused. Java is slightly older. Javascript chose the name to capitalize on the popularity.
Of course, many backend developers look down on Javascript. They believe it to be inferior.
Javascript has come a long way. From its early beginnings, it is now more feature-rich. Things like AJAX, jQuery, and node.js have brought great strides.
Compare
Learning something new can be done through comparison. Java and Javascript have similar features. Let’s first look at two code examples.
Here is a Javascript function to sum two integers.
function sum(x, y) {
return x + y;
}
Here is a Java method to sum two integers.
private static int sum(int x, int y) {
return x + y;
}
These two examples look pretty similar with subtle differences. Notice the Javascript function doesn’t mention type. Where Java requires it. This was one thing I remember stumbling upon initially.
Typing
This is sometimes referred to as strong vs weak typing. This question from StackOverflow explains it in detail. Typing is a differentiator between the two.
Compiler
Java is a compiled language whereas Javascript is not. Interpreted languages like Javascript to bring different challenges. For example, the compiler will catch some errors.
Usage
Java has made a name for itself on the server, primarily used for backend development. I first encountered Javascript as a tool to check form input on web pages.
Frameworks
Software development has taken a big step forward with frameworks. On the Java side, we first had Struts then came Spring. Javascript has jQuery, Angular, and React.
These foster reusable code. Instead of everyone creating a different utility to check for a valid email address. We can use one that is well-tested and open-source.
jQuery
Having worked with jQuery a few times this is a quick example. It has been around for a while and is useful.
<html>
<head>
<title>Trying out jQuery</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width">
<script type="text/JavaScript"
src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js">
</script>
</head>
<body>
<h1>jQuery Example</h1>
<script type="text/javascript">
$(document)
.ready(
function() {
$("button").click(
function() {
$("#bClick").html("<h2>Clicking</h2>");
});
});
</script>
Click the button to see demo
<button>Click me</button>
<div id="bClick"></div>
</body>
</html>
Spring
Spring Boot helps you create an application easily. Coupled with the Spring Initializr a few clicks and you have an app.
package co.codeiseasy.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Mixing frameworks from both languages helps you create robust applications quickly.
Learning
Once I got serious about learning Javascript I began with this free course from CodeAcademy. It covers a lot of basic stuff. I have been dabbling in Javascript for years. However, it has changed a lot.
Another great site is LearnJavascript. Jad Joubran put this together to help people learn the fundamentals. It even has flashcards you can get on your phone to reinforce the learning.
Overall, in my career, I have been in and out of Javascript. Recently I climbed back in with Vue.js. I learn something new from each interaction.
For many years I tried to go deep into Java. Although learning more about Javascript and other languages gives you a new vantage point. Similar to speaking different languages, you understand it better.
One last point I would make is this. Learning something new is humbling. It reminds me of the Shoshin. This is from Zen Buddhism—the idea of having an open and eager mind.
Keep an open mind and keep learning.