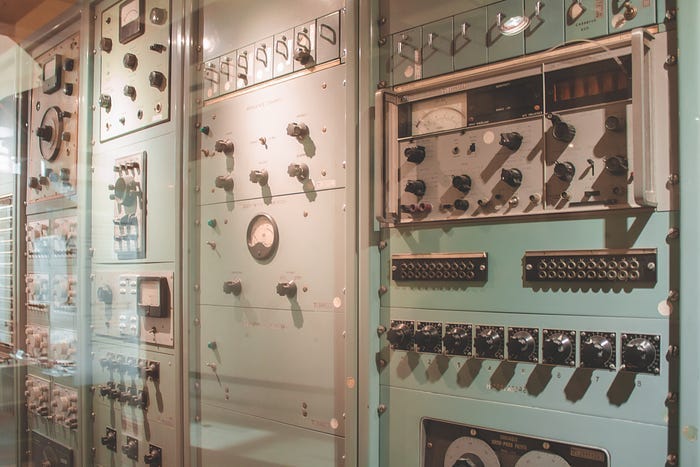
The first programming I did with the Atari 400 was cumbersome. I worked through some examples to understand the BASIC programming language. Vue.js is easier to learn but has a broader API.
I had to spend countless hours, above and beyond the basic time, to try and perfect the fundamentals.
Julius Erving
The time spent programming was enlightening. Each mistake in BASIC or Vue.js reveals new insights. Success or failure can help you down the road. Let’s learn a little more in Vue.js.
Emits
Our sons' basketball practice never ended on time. So he would usually text us to pick him up. Likewise, child components need to notify the parent component. This is done through an emit() event.
<script setup>
import { ref } from 'vue'
import ChildComp from './ChildComp.vue'
const childMsg = ref('Not done yet.')
</script>
<template>
<ChildComp @response="(msg) => childMsg = msg" />
<p>{{ childMsg }}</p>
</template>
Here is the parent component. It is set to receive the emit() event from the child component.
<script setup>
const emit = defineEmits(['response'])
emit('response', 'We are done. Can you pick me up?')
</script>
<template>
<h2>Son at basketball practice</h2>
</template>
This is our ChildComp.vue that sends the message “We are done. Can you pick me up?”. This updates the childMsg on the Parent.vue.
The simple example has the message updated on the parent component.
Slots
We have passed props and emit messages. If you want to pass template fragments you can use slots.
<script setup>
import { ref } from 'vue'
import MartianComp from './MartianComp.vue'
const msg = ref('take me to your leader!')
</script>
<template>
<MartianComp>Martian Message: {{ msg }}</MartianComp>
</template>
In this main component, we use the MartianComponent. This our slot we can pass the code.
<template>
<slot>Martian content</slot>
</template>
The MartianComp has the slot to receive the code. Emit and slots are similar tools. Depending on your use case experiment to see which one works best.
Progressive Framework
We have come a long way. Let’s step back to review where Vue.js fits in the bigger picture. It is part of a larger ecosystem. Frontend development can be demanding, so you need to integrate other technologies.
Vue is designed to flex in many ways. As your stakeholders ask for changes you can adapt with it. With basic HTML and JavaScript skills, you can harness the power of the web.
These last bits should help you see what you can do. The basics whet your appetite for future experiments. Create an application and try this out. Also, read some documentation to fill out your understanding.